PHPで画像に余白を付けるスクリプト[正方形]
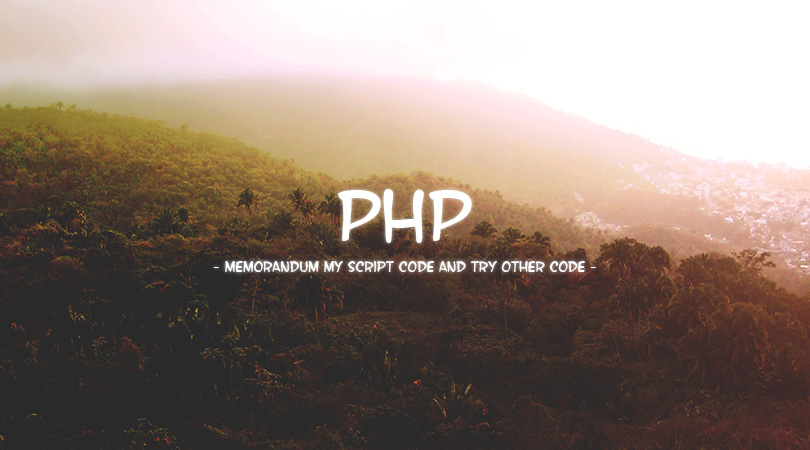
いきなりマニアックな記事だけど、仕事で作ったので備忘録。
どういうスクリプトか
まずどういう事が出来るのかを書く。
画像名を設定して、画像ファイルと幅と余白を渡す。するとその幅その余白内に縮小された画像を返す。
つまり、
こういう事になる。これは幅600pxで余白60pxに設定した場合。
入れる画像が正方形でない場合、長い辺に合わせて縮小される。
今回はクラスで作った。使い方はまず、呼び出して名前を設定。
require_once( 'models/ImageMake.php' ); // ファイルを呼び出す。オートローダー機能がある場合は不要 $makeImage = new ImageMake(); // オブジェクトをインスタンス化する $makeImage->setName( $name ); // setName関数で名前を設定
そして画像ファイルをmakeSquare関数に渡す。
if( isset( $file )){ // $fileは$_FILESで受け取った画像ファイル $makeImage->makeSquare( $file, 600 , 60 ); // 引数は 画像ファイル , 幅 , 余白 }
これで上記のファイルが指定のフォルダに保存される。
ちなみに画像名、保存先パスはデフォルト値を設定可能なので、適宜置き換えてください。
中身
クラスの中身。
class ImageMake { protected $name = 'default_name'; // 画像名 protected $path = "/img/"; // 保存先パスはドキュメントルート以下を設定。スラッシュ必要 public function setName( $name ) { $this->name = $name; } public function setPath( $path ) { $this->path = $path; } /*-------------------------------------------------- 正方形を作る * $file : 画像ファイル * $length : 作成後の画像サイズ * $white : 作成後画像内の余白 * return : JPEG画像ファイル / false --------------------------------------------------*/ public function makeSquare( $file , $length = 600 , $white = 60 ) { $make_width = $length - $white * 2; // 画像の幅と高さを算出 $make_height = $length - $white * 2; $path = $_SERVER['DOCUMENT_ROOT'] . $path; // 保存先のパス /*-------------------------------------------------- 1、画像整形 --------------------------------------------------*/ $image = $file['tmp_name']; list( $org_width , $org_height ) = getimagesize( $image ); // 元画像のサイズを取得 $ratio = $org_width / $org_height; // 縮小の倍率を計算。長い方を基準に縮小 if ( $make_width / $make_height > $ratio ) { $make_width = $make_height * $ratio; } else { $make_height = $make_width / $ratio; } $image_created = imagecreatetruecolor($make_width, $make_height); $image = imagecreatefromjpeg( $image ); imagecopyresampled( $image_created , $image , 0 , 0 , 0 , 0 , $make_width , $make_height , $org_width , $org_height ); // 縮小された元画像 /*-------------------------------------------------- 2、背景画像を生成 --------------------------------------------------*/ $base_img = imagecreatetruecolor( $length , $length ); $bg_color = imagecolorallocate( $base_img, 255, 255, 255 ); imagefilledrectangle( $base_img , 0 , 0 , $length , $length , $bg_color ); // 白の背景を$lengthのサイズで作成 /*-------------------------------------------------- 3、1と2をを合成 --------------------------------------------------*/ imagecopy( $base_img , $image_created , ( $length / 2 ) - ( $make_width / 2 ) , ( $length / 2 ) - ( $make_height / 2 ) , 0 , 0 , $make_width , $make_height ); $name = $this->name . $length . '.jpg'; if( imagejpeg( $base_img , $path . $name , 100 )){ // 合成した画像を保存先パスに移動 return $base_img; } else { return false; } } }
今回は600px幅、60px余白で作っているので、元画像の大きさは480pxになる。( 600 – ( 60 * 2 ) )
1の部分で元画像を480pxの画像にする。
/*-------------------------------------------------- 1、画像整形 --------------------------------------------------*/ $image = $file['tmp_name']; list( $org_width , $org_height ) = getimagesize( $image ); // 元画像のサイズを取得 $ratio = $org_width / $org_height; // 縮小の倍率を計算。長い方を基準に縮小 if ( $make_width / $make_height > $ratio ) { $make_width = $make_height * $ratio; } else { $make_height = $make_width / $ratio; } $image_created = imagecreatetruecolor($make_width, $make_height); $image = imagecreatefromjpeg( $image ); imagecopyresampled( $image_created , $image , 0 , 0 , 0 , 0 , $make_width , $make_height , $org_width , $org_height ); // 縮小された元画像
2ではただ白い600pxの画像を生成。これを背景に敷く。
/*-------------------------------------------------- 2、背景画像を生成 --------------------------------------------------*/ $base_img = imagecreatetruecolor( $length , $length ); $bg_color = imagecolorallocate( $baseImg, 255, 255, 255 ); imagefilledrectangle( $base_img , 0 , 0 , $length , $length , $bg_color ); // 白の背景を$lengthのサイズで作成
3で1と2を合成。2( $base_img )の上に1( $image_created )を足す感じ。
/*-------------------------------------------------- 3、1と2をを合成 --------------------------------------------------*/ imagecopy( $base_img , $image_created , ( $length / 2 ) - ( $make_width / 2 ) , ( $length / 2 ) - ( $make_height / 2 ) , 0 , 0 , $make_width , $make_height ); $name = $this->name . $length . '.jpg'; if( imagejpeg( $base_img , $path . $name , 100 )){ // 合成した画像を保存先パスに移動 return $base_img; } else { return false; }
だいたいこんな感じ。
このスクリプトは画像のリサイズとかから応用したんだけど、そういうのは他のブログにもよく書かれているので今回はちょっと変わった使い方のほうを記事にした。
一応長方形の関数もあるんだけど、今回は正方形ということで。また時間があれば(もしくは要望があれば)書きます。